Introduction to R
This workshop is beginner-level introduction to programming in R. The course is designed to be taught in two sessions of 3 hours each and is focused on the application of R to the analysis of tabular data from clinical trials.
R Markdown File
R Markdown files act as notebooks which can make it easier to document and share code. All the results from the code as well as your comments and descriptions can be combined into one, tidy document. This makes it easy to combine insights, protocols and figures in a practical and organized fashion.
Learning objectives
Basic Operations in R
R Markdown vs. R Script
RStudio IDE
Arithmetic Operations
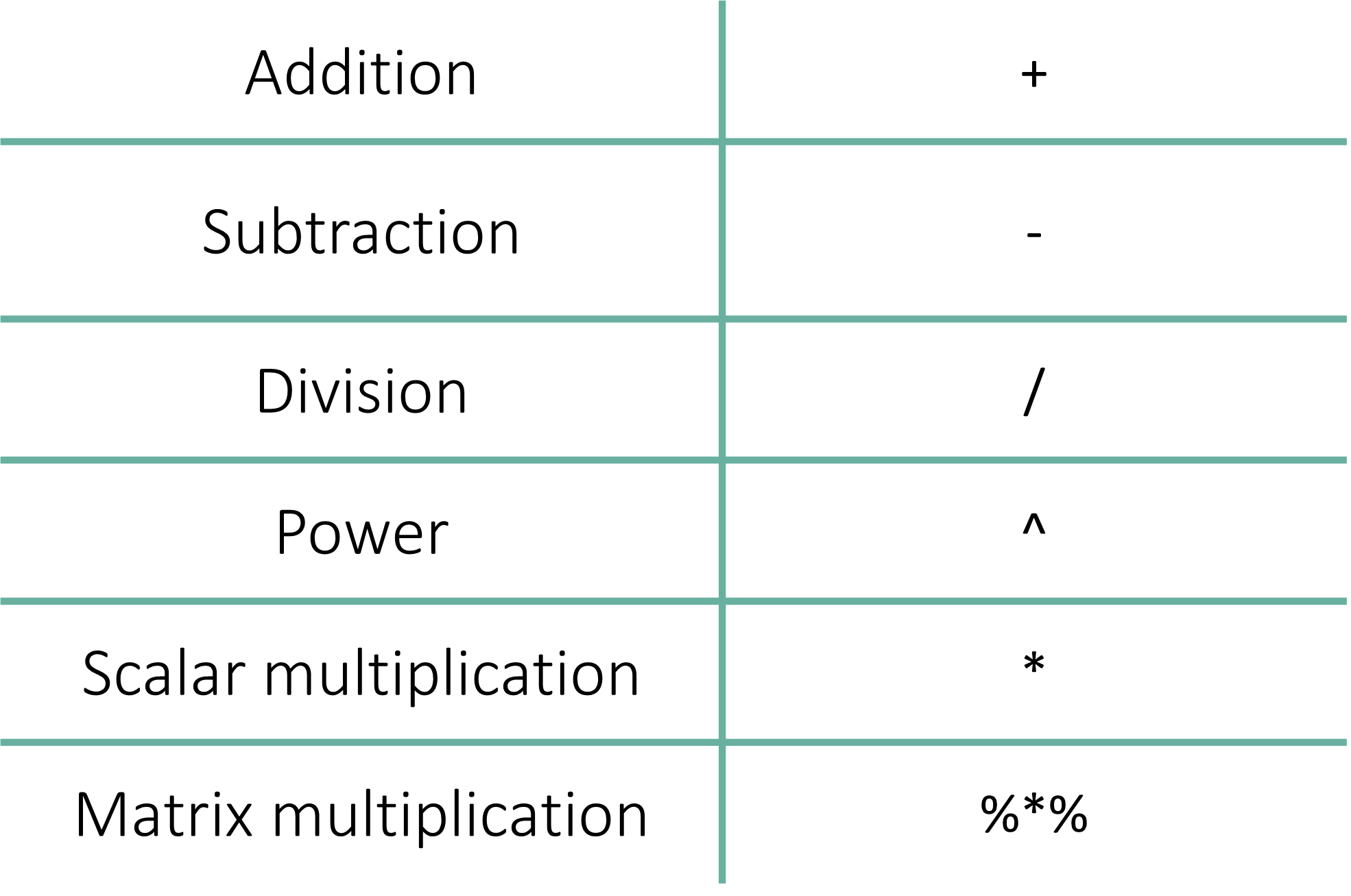
## [1] 100002
## [1] -2
## [1] 639
# Division
90/((3*5) + 4)
## [1] 4.736842
## [1] 8
Syntax
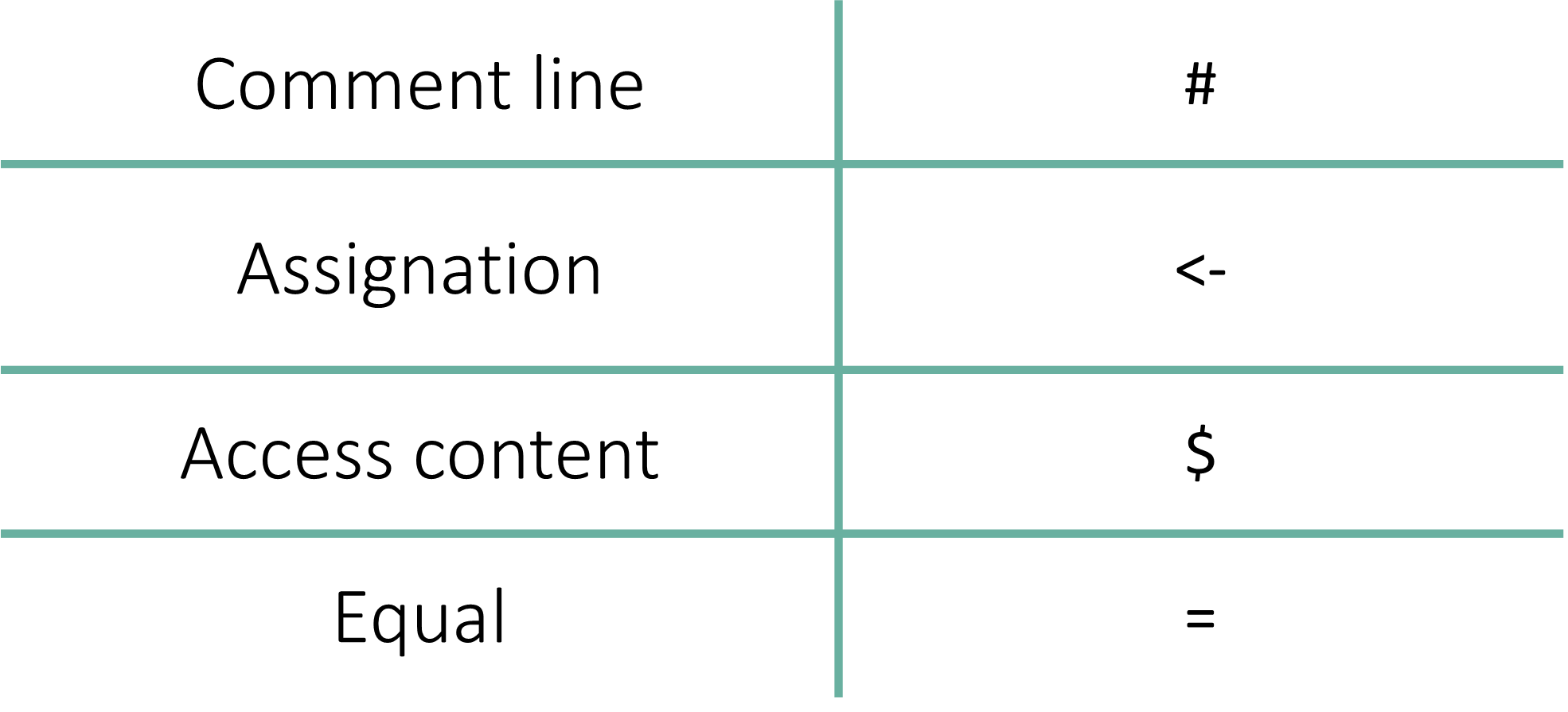
Creating variables
# The convention is to use left hand assignation
var1 <- 12
var2 <- "hello world"
Printing Variables
## [1] 12
## [1] "hello world"
## [1] 12
## [1] "hello world"
# It is also possible to use the '=' sign, but is NOT a good practice
var1 = 13
var2 = "hello world"
var1
## [1] 13
## [1] "hello world"
Logical operators
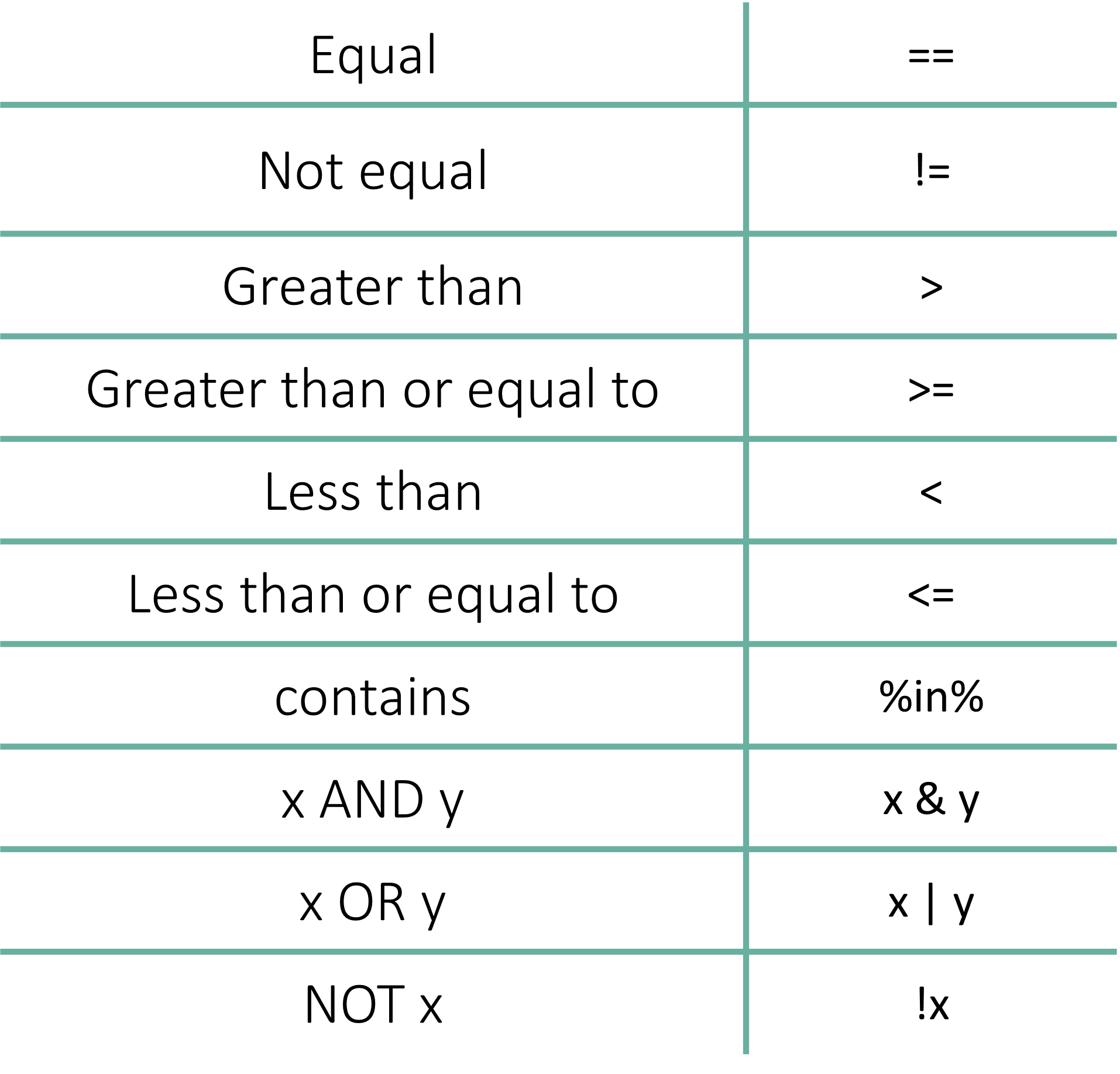
# First create two numeric variables
var1 <- 35
var2 <- 27
## [1] FALSE
# Less than or equal to
var1 <= var2
## [1] FALSE
## [1] TRUE
# They also work with other classes
var1 <- "mango"
var2 <- "mangos"
## [1] FALSE
Strings are compared character by character until they are not equal or there are no more characters left to compare.
## [1] TRUE
We can test if a variable is contained in another object
## [1] TRUE
## [1] FALSE
Exercise
- Write a piece of code that stores a number in a variable and then check if it is greater than 5. Try to use comments!
- Bonus: Is there a way to store the result after checking the number?
## [1] TRUE
## [1] TRUE
2.3 Comments
Comments are embedded into your code to help explain its purpose. This is extremely important for reproducibility and for documentation.